I’m currently making a RPG game in java, and have been thinking of moving on to multithreading. But I don’t know what I should use, thread spawning or thread pool. Which one should I use? and why?
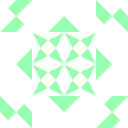
I’m currently making a RPG game in java, and have been thinking of moving on to multithreading. But I don’t know what I should use, thread spawning or thread pool. Which one should I use? and why?
Why do you want to use multiple threads? Any specific reasons? Using multiple threads is a bad idea in games and it leads to many problems.
Please stay on topic, this thread is about what type of mutlithreading I should use, not why I do it.
That’s about as on topic as you can be. There’s obviously no point asking for multithreading solutions if you don’t need it in the first place. Multithreading isn’t like implementing game mechanics; you don’t implement it when you want, you implement it when you need it.
That’s about as on topic as you can be. There’s obviously no point asking for multithreading solutions if you don’t need it in the first place. Multithreading isn’t like implementing game mechanics; you don’t implement it when you want, you implement it when you need it.
This isn’t a topic about which threading i should use. I specifically as for what type of multithreading is best for a game. Even if none of them are better than single threading. Please let me struggle, and fail if I must. At least I learn exactly why it shouldn’t be used.
Your original question does show that you may be lacking when it comes to experience, so it’s understandable that people would discourage you. Multithreading is very complicated to get right.
To actually answer your question: When it comes to games (or when performance is extremely important in general) you should avoid creating expensive objects each frame. New instances eventually add up and the garbage collector has to be run. When it comes to threads however you should avoid creating lots of them not because of the garbage but because threads are relatively expensive to create. You may also encounter slightly weird timings since it may take a relatively long time to start a new thread, so creating a new thread each frame to run a specific task is generally a bad idea. Pooling threads and distributing tasks to them using a blocking queue has better and (more importantly) more predictable performance.
Might as well add a shameless plug… I’m developing a threading library for games which allows you to split your game up into individual tasks and then set up complicated dependencies between these tasks. The library will then identify which tasks that can be run in parallel and distribute them to the threads in a thread pool. I suspect that this isn’t exactly what you’re looking for, but it can help you skip the boring thread management and let you focus on learning about multithreading games and synchronization if that’s what you’re after.
You won’t get any better feedback unless you tell us what you are planning to use the threads for. Asset loading in background? Separate thread(s) for socket/multiplayer? etc…
At least I learn exactly why it shouldn’t be used.
Easy.
Do these in order!!
If you really want to use multithreading, first rule is to learn beginner’s FAQs on multithreading:
Can Someone Explain Threads to Me?
How do you kill a Thread in Java?
What is a daemon thread in Java?
How to timeout a thread
How can I pass a parameter to a Java Thread?
How do synchronized static methods work in Java and can I use it for loading Hibernate entities?
Why is creating a Thread said to be expensive?
Do spurious wakeups in Java actually happen?
How to make a Java thread wait for another thread's output?
Will Threads Make My Game Run Consistently On Every Computer
Second rule, learn about differences:
"implements Runnable" vs "extends Thread" in Java
Difference between wait() and sleep()
What is the difference between a process and a thread?
Java: notify() vs. notifyAll() all over again
Is there an advantage to use a Synchronized Method instead of a Synchronized Block?
difference between synchronizing a static method and a non static method
Java concurrency: Countdown latch vs Cyclic barrier
Java Timer vs ExecutorService?
Seeking Clarity on Java ScheduledExecutorService and FutureTask
Third rule, how to apply what you’ve learned:
What are the advantages of using an ExecutorService?
How to implement a fixed rate poller with ScheduledExecutorService?
How to handle errors in a repeated task using ScheduledExecutorService?
Fourth rule, understanding how to handle exceptions thrown by these multithreaded problems:
java thread exceptions
Java-Exception Handling
Handling exceptions from Java ExecutorService tasks
ConcurrentModificationException for ArrayList
Deleting objects from an ArrayList in Java
And finally, fifth rule, what problems can be caused by multithreading:
Problems with Singleton Pattern
Multi-threading and Java Swing Problem
Producer/consumer multithreading
How to improve performance on two thread accesing a collection of items concurrently
After completing the sessions from above, you’ve learned multithreading and have at least a professional-looking background experience.
Additional info on multithreaded unit testing, something that I think it’s worth it look at.
You should probably thank me for compiling all of these informations that were provided by StackOverflow.
Threads are fascinating and multithreading will only become more valuable as multicore cpus become more prevalent. However, experimenting with them could very likely lead one into writing a game that is unlike, in some fashion, the thousands of clones currently being generated by game engine programmers, and we can’t have that, can we??
I’d put the question in two stages. The first is whether the cost of managing either spawning or pooling is justified to begin with. That is where most of the answers seem to be landing in the negative for “traditional” game applications. Perhaps they haven’t considered this: “No More Callbacks: 10,000 Actors, 10,000 Threads, 10,000 Spaceships” http://blog.paralleluniverse.co/
The second stage probably can be related to general tradeoffs about spawning or pooling in general. Spawning has more overhead per unit spawned (creation, garbage collection). Pooling has an up-front overhead greater than spawning (building the pool), but once the pool is created, managing the objects can potentially be less overhead since creation and garbage collection are avoided.
So, it is kind of like a classic breakeven analysis with “fixed” and “variable” costs. Spawning has more variable cost, pooling has more fixed cost.
A lot will depend on your intended use. How long will the thread run? How many threads at once? How much reuse are you looking at? Brian Goetz (“Java Concurrency in Action”) points out that frequent, light-weight use of threads creates significant costs in building and tearing down the threads. If that is your scenario, then pooling is indicated.
If the threads will get a lot of use once made, and will be built infrequently, maybe spawning is better.
I don’t know if there is a way to determine this except by trying and experimenting and profiling.
However, Brian Goetz does give this equation for tuning the size of a thread pool (in “Java Concurrency in Practice”, 8.2):
N = number of cpus
U = target cpu utilization, 0 <= U <= 1
W = wait time
C = compute time
The optimal pool size for keeping the processors at the desired utilization is:
Threads = N * U * (1 + W/C)
The point is that one thread per cpu makes a certain sense, but if the threads spend a lot of time in a blocked state, it might make sense to use more threads than there are cpus. But Goetz also points out there may be other constraints affecting the best number of threads in a pool, such as external resource availabilities.
I hope all this adds something to the discussion, even if it isn’t a clear rule-of-thumb answer.
Old Discussion on Game Threads
Okay, completely off-topic, but it does help to know that the history of games and creating multiple threads doesn’t go without animosity.
Thread Spawning vs. Thread Pooling
For games, thread spawning always works better for me. Whenever I use a thread pool, it is just cumbersome for games because you have better things to manage than an array of thread workers eating up CPU time for nothing. In other words, there is really no reason in games I can think of where a constant running thread pool would yield an advantage.
You have to think about, when a user will be using this?
Probably only during the main game…
How many things in my games need a separate thread?
Usually, not many and the thread work is usually finite.
Especially in an RPG, I already know that there is probably nothing that CPU intensive anyway. Did you really want the CPU running when your user decides to pause the game? Really?
In games, you want to use as much resources as you can to get the data to the screen. All this fancy threading stuff, leave that for cell generation demos, or space eruption simulators. A game should be focused on user enjoyment, not technical stuff that eat CPU time for no reason. Anyway, since you were very broad with what you were building (a simple RPG), this answer will suffice. If you are more specific on what you need all these threads for, maybe then we can give a better solution.
Multi threading is useful for large tasks that would hold back the rest of your program. If you are going to use it you need to make sure that the threads that rely on each other are coordinated so. You will also need to make sure they are not created when you make an object such as creating a new thread when you make a player this can overload your computer and is a common mistake with threading.