Here is a picture of what my Sun looks like (don’t mind the black triangles…those are other errors I had in code and not related to the Sun, they were related to my lighting and object positioning distances.)
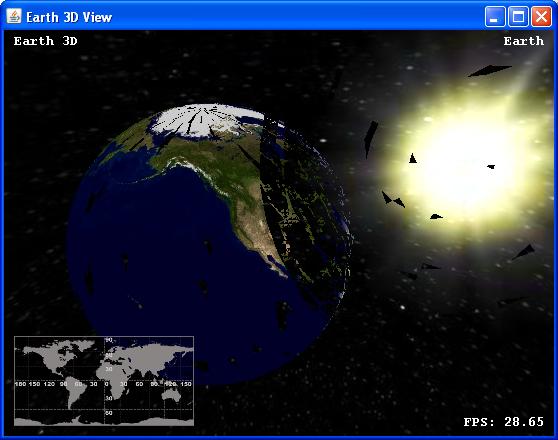
And this is the code that I managed to write to get me pretty good blending…I used a gluDisk because it is round and I put the texture on it…when you add blending it makes the dark part around the Sun’s rays look transparent enough to fool the eye pretty well (notice how the stars in the background are visible through the Sun’s rays)…I think it looks good.
// Draw disk with texture of stars on the inside
gl.glPushMatrix();
gl.glScalef(1.0f, 1.0f, 1.0f);
gl.glDisable(GL.GL_LIGHTING);
gl.glEnable(GL.GL_TEXTURE_2D);
gl.glRotatef(90.0f, 0.0f, 0.0f, 1.0f);
gl.glBindTexture(GL.GL_TEXTURE_2D, textureId);
gl.glEnable(GL.GL_BLEND);
gl.glBlendFunc(GL.GL_ONE, GL.GL_ONE);
gl.glDisable(GL.GL_CULL_FACE);
glu.gluDisk(sunBillboard, 0.0f, 50000.0f, 32, 32);
gl.glDisable(GL.GL_BLEND);
gl.glEnable(GL.GL_CULL_FACE);
gl.glPopMatrix();
There might be some useless calls in there but I’ve not had time to clean it up. My gluQuadric is the ‘sunBillboard’ and the ‘textureId’ pertains to the sun texture I had. I also wrote code that determines where your eye is at with respect to the location of the Sun and it keeps rotating the Sun around to face you (ie the billboard effect).
So, in my case using the GL_ONE blending seemed to work…it might also have to do with how your scene is setup with lights, etc…I wouldn’t actually know, I’m just speculating.