Fixed-time-step means the same thing as discrete time. Discrete means you can have values of 0, or 1, or 2, and so on, but not 0.5, 1.234, or 2.0001.
[quote=“hvince95,post:14,topic:40303”]
I want to predict the time in the future where a collision occurs. I do not want to save old x/y positions/velocities or time stamps. I have an update function that moves an object with an x-velocity, y-velocity, and position. The position and velocity of an object at time t would be a function of only its state at t-1. Not a function of t relative to the beginning of time or relative to some arbitrary time stamp from the past.
Predictions, on the other hand, need to be valid given a function of t and the present velocity/position with t = zero being the present.
[quote=“hvince95,post:14,topic:40303”]
It wasn’t tedious, though you did use frightening notation (such as y’). One of the problems with your post is that you use a parabolic equation as the basis of your equations. Given the version of the update function I had at the time (y += vx;
), a real parabola doesn’t give the same results.
y’ = (u * t + 1/2 * gravity * t2) + yi
y = 1/2 * gravity * t2 + v * t + y0 = f(t)
Let g = -1, v = 2, y0 = 3.
[tr][td]f(0) = 3[/td] [td]y0 = 3[/td][/tr]
[tr][td]f(1) = 4.5[/td] [td]y1 = 5[/td][/tr]
[tr][td]f(2) = 5[/td] [td]y3 = 6[/td][/tr]
[tr][td]f(3) = 4.5[/td] [td]y3 = 6[/td][/tr]
[tr][td]f(4) = 3[/td] [td]y4 = 5[/td][/tr]
So… it looks like a parabola, but it’s not. The equivalent equation h(t) that passes through the points yn is already posted here. The step-by-step simulation needs to be the same as the prediction function. However, now I think I can replace yn+1 = yn + vyn with yn+1 = yn + vyn + gravity / 2. And then both processes will give the same results. I’ve done the math for both the parabola and arithmetic series equations (and found t is a little simpler to calculate directly using a parabola than an arithmetic series,) but I’m not done yet.
I’m left with the following considerations:
- How expensive is a square root relative to multiplication?
- If finding t directly is slower (it is for anything besides flat horizontal platforms), should I drop the requirement optional condition or find t in two steps (prune the relatively large amount of bad trajectories and only calculate t for the remaining ones)? *
- How can I apply this to more complicated structures, like walls, ceilings, or slopes? **
-
b[/b] If the object is assumed to travel in an arc for the prediction, how should I ensure actual collision detection in the step by step method?
- This is extremely specific to my application, so I don’t expect an answer for this. I’m just writing it to summarize my train of thought on this. - I may drop this requirement mainly because I discovered I can apply my prediction method, no matter what it turns out to be, to other objects, including hills, walls, ceilings, solid blocks, AABB based targets, and moving platforms (but not necessarily with precise collision times). It would have been very helpful if only the simplest case (as described in my first post) were possible, but it’s not really needed as an “optimization” anymore. Though it still could help for something else.
** This can be done by looking at the y position of the particle before an after it passes by an object (assuming it goes through it). It’s sort of like an intersect function for rectangles. For example, collision with a wall is simply f((wallX - x) / vx) > bottom && f((wallX - x) / vx) < top… So I’ve solved this on my own, too, slightly differently than my original thoughts.
The last part is currently my biggest concern. If the predicted collision time for a simple vertical platform was 10.5 for example, the positions of the object at times t = 9 and t = 10 where on opposite sides of the platform but didn’t individually intersect with it, then what method should be used to detect collision in the actual step by step process?
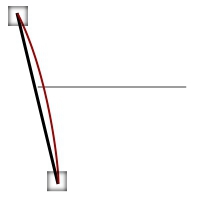