Playing around with http://hexara.com/SimplexVisualizer.html I got the following, which seems pretty close.
http://www.java-gaming
.org/user-generated-content/members/27722/jgo-reply.jpg
Here is a screen shot of the settings I used:
Basically, what @LiquidNitrogen and @CptSpike is correct. The noise() function returns values between -1 and 1, and we want to scale the results to something like 0 to 255 for graphics output. The method of scaling used for your example includes an absolute value. This form of mapping was termed “Turbulent Noise” by Ken Perlin.
For example, (pseudo code)
for ( all y values)
for ( all x values)
noiseValue = noise(x * xScaling, y * yScaling);
noiseValue = Abs(noiseValue) * 255;
postValueToImagePixel(x, y, noiseValue);
A “Smooth Noise” mapping would be more like the following:
noiseValue = ( (noiseValue + 1) / 2.0 ) * 255;
I don’t know about your particular noise() function, or why you are putting 4 parameters in it. With Gustavson’s Simplex Noise implementation, you only need the X & Y for 2D noise.
Your pattern might have a bit of “clamping” to it on the black end. That can be accomplished by something like the following:
noiseValue = abs(noiseValue); // applies 'turbulent noise' algo
noiseValue = ( noiseValue * 1.2 ) - 0.2; // scale and shift
noiseValue = max(0, noiseValue); // "clamp" levels below 0 to 0
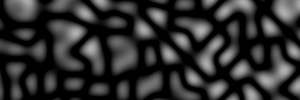
Another possibility is that the multiplication in the second step above is omitted, and the values simply shifted downward with the subtraction, then clamped. I say that because it seems to me the results don’t get as bright as I expect at the white end, so maybe the shift was uniform across the entire response range, rather than being “stretched” by the multiplication first.
There may be a second noise function being summed in, in which case the noiseValue starts out as the weighted sum of the two calls to the noise() function, before going through the various transforms to make the number an intelligible color value.
You can download the jar of Sivi from here:
http://www.java-gaming.org/user-generated-content/members/27722/sivi.jar
Or, check out the github open source here: