Thanks for the reply,
The padding-error.png picture looks exactly like my issue.
I am using the Nearest filter on every Texture.
I followed the first link and ran into a bunch of other links I have attempted. The first time I encountered this I thought it was a floating point division issue. I tried recasting everything either way, and I also printed out all of the x,y,h,w data for each Sprite/Texture.
I manually calculated each height, width, x, and y and according to the values everything should look perfectly square. This is why I think it is an OpenGL issue.
Here is a picture and the code that produced it:
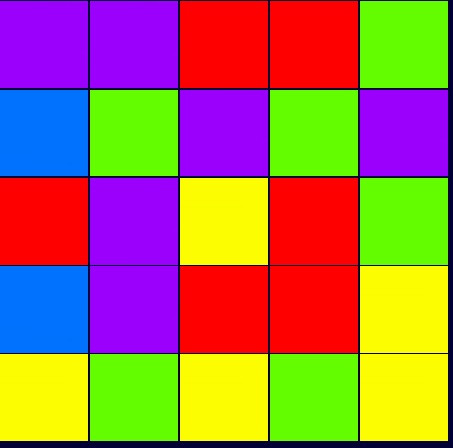
public void create() {
camera = new OrthographicCamera();
camera.setToOrtho(false, 550, 550);
batch = new SpriteBatch();
spriteContainer = new Array<Texture>();
redTexture = new Texture(Gdx.files.internal("data/red.png"));
greenTexture = new Texture(Gdx.files.internal("data/green.png"));
blueTexture = new Texture(Gdx.files.internal("data/blue.png"));
yellowTexture = new Texture(Gdx.files.internal("data/yellow.png"));
purpleTexture = new Texture(Gdx.files.internal("data/purple.png"));
redTexture.setFilter(TextureFilter.Nearest, TextureFilter.Nearest);
greenTexture.setFilter(TextureFilter.Nearest, TextureFilter.Nearest);
blueTexture.setFilter(TextureFilter.Nearest, TextureFilter.Nearest);
yellowTexture.setFilter(TextureFilter.Nearest, TextureFilter.Nearest);
purpleTexture.setFilter(TextureFilter.Nearest, TextureFilter.Nearest);
colorContainer = new Array<Texture>();
colorContainer.add(redTexture);
colorContainer.add(greenTexture);
colorContainer.add(blueTexture);
colorContainer.add(yellowTexture);
colorContainer.add(purpleTexture);
createSpriteContainer();
}
@Override
public void render() {
Gdx.gl.glClearColor(0, 0, 0.2f, 1);
Gdx.gl.glClear(GL10.GL_COLOR_BUFFER_BIT);
camera.update();
batch.setProjectionMatrix(camera.combined);
batch.disableBlending();
batch.begin();
for(int x = 0; x < 8; x++)
{
for(int y = 0; y < 8; y++)
{
batch.draw(spriteContainer.get(x + y * 8), 20 + x * 65, 20 + y * 65);
}
}
batch.end();
}
private void createSpriteContainer()
{
for(int x = 0; x < 8; x++)
{
for(int y = 0; y < 8; y++)
{
spriteContainer.add(colorContainer.random());
}
}
}
I created the tiles by just changing the color of the tile to each of the colors and exporting. So the tiles should all be identical except for the color.