I’m attempting to figure out how I can add lights to my current game, it’s top down 2d and there need to be multiple instances of the lights that are moving. I’ll focus on making them dynamic at another date. But, I have no idea how i would do this with libgdx. Any further details can be provided at request. Any help or suggestions is greatly appreciated.
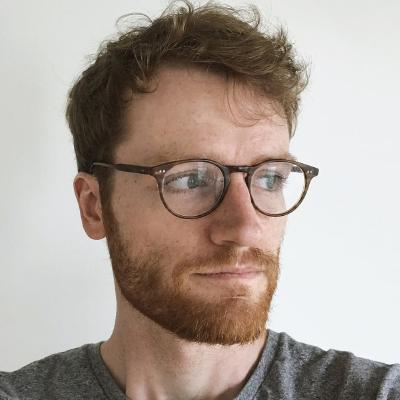
mattdesl/lwjgl-basics
:wrench: LibGDX/LWJGL tutorials and examples. Contribute to mattdesl/lwjgl-basics development by creating an account on GitHub.