I do something similar.
For example, for humanoid characters I have the body sprite, the head sprite, and the hands (or weapons) sprite, each set to an specific position and rendering order.
All I have to do is swap the actual bitmaps around. Of course all bitmaps of the same type (say, head sprites) are drawn using the same constraints (size, position, etc).
Here you have an example, except for the scientist in the middle, all these use the same body sprite with different colors, and all I do is swap the head sprites:
As for the armour, I just draw a heavier-looking torso and shoulder pieces on top of the same body.
I recommend you try to make sprite composition script based. For example, make a parser that reads a simple text file with all the information regarding sprite position, bitmap names, animation frames, etc… That way you won’t have to delve into code whenever you want to modify the content.
XML Example just off the top of my head (not actual code):
<sprite name="Jhonny">
<subsprite name="Head" xPosition="0" yPosition="10">
<file>/sprites/JhonnyHead.png</file>
<animation name="RUN">
<frames>1,2,2,3,2,2,1</frames>
</animation>
</subsprite>
<subsprite name="Body" xPosition="0" yPosition="0">
<file>/sprites/JhonnyBody.png</file>
<animation name="RUN">
<frames>1,2,3,4,5,6,7</frames>
</animation>
</subsprite>
</sprite>
In fact, the way I have it set up, I can have sprites with mismatching frames, and make them match through the script. For example, the feet’s walk animation has more frames than the shoulders, yet through the script I instruct the animation class to reuse specific frames so they match.
A cute error I’m dealing with now is that the different body parts get out of synch, here you can see an example:
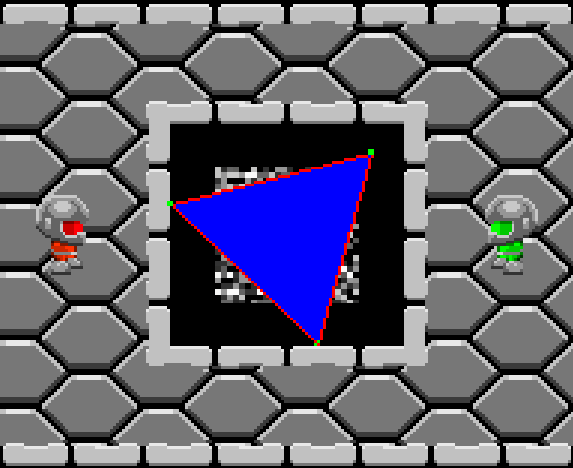
Notice how the head bounces weirdly? Part of the animation script instructs the sub elements how they need to bounce (so I don’t need to include the bounce in the actual sprite, in fact the head parts only have one frame), which is another way to avoid making more sprites than needed.
In any case, just a bunch of ideas, you’ll find the solution that best matches your needs 