Oh, I have no aspirations of changing the Java community, lol. Mostly, its just a chance of trying to make something for the public instead of by myself, and to get some feedback on what I’m doing. However, I do genuinely feel that this would be a good library for some one to start writing games with.
So, the central class of my library is Game, it handles the JFrame and canvas (which I suppose means this is based on Java2D), and is where the main loop is run. Each loop, Game updates and draws the current screen (a Holder defined by the programmer) which starts a chain reaction down components.
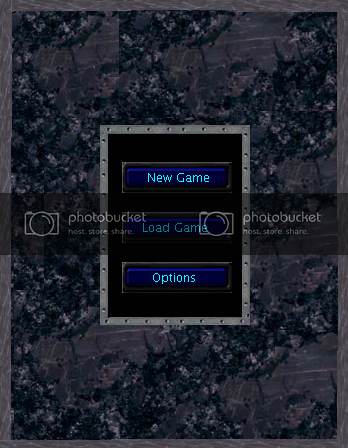
The above is the MainMenu screen of my project*, Game sends the update() and draw() commands to MainMenu which in turns sends them to the inner Holder (w/ buttons) which in turns sends them to the Buttons. This way, people can plug in new screens (thus games) without having to alter Game, and most things are accomplished just by overriding update(). Game uses a similar method to cascade MouseEvents to the components to which the events apply, which is how the Buttons alter their state.
Each Holder can hold any number of other GUI elements, including other holders, as in the following. Three holders (status, and console, and world) are added to the screen, which contain other components. The first two are just typical holders, but World is a special holder to work with Entities (which are still derived from the same parent as other components), for those games that use entities. A zIndex of objects used by the Holders allows drawing order to be changed, so for example a unit can go under and over a bridge, its also useful in simple GUI like placing the console over the world.
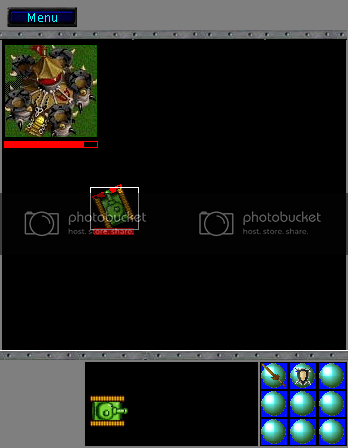
Another benefit is the easy alteration of game time, for opening menus, dialog, etc. To pause the game, I just have to shift a quickly defined activeObject from the screen itself to the menu (etc.).
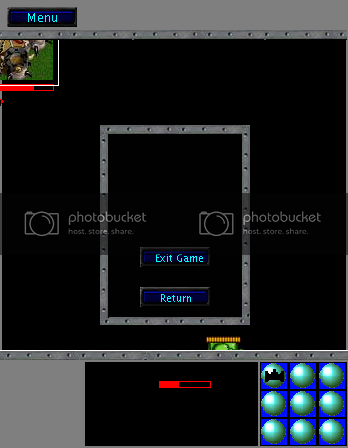
That’s pretty much the basis of it. I mean, I can throw together new screens in a few minutes, and theres already plenty of objects to build interfaces for various game designs. Would this be a useful library?
Update:
Got Tetris back up, same design. A main Holder contains several Holders and uses update(), this also uses the Grid class, which will be good for board games (probably as a subclass)
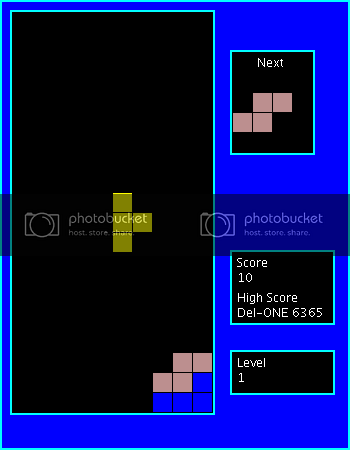
Now that I think about it I could probably make it so that I can move between games within a single session by just defining a new Screen.
P.S. I know this sounds horrible, but what are widgets?