OK, I will give you a quick example, but I will also explain myself a bit which I think will help more. And sorry, I forget that pretty basic mechanics generally aren’t taught in schools (in England I had to do an A-Level in physics to be taught about angular velocity and even then it was only very basic).
Angular velocity is a bit of a hard thing to get your head around if you aren’t used to it. So you can think of velocity as the speed and direction in which position is changing (rate of change of position if you’re big on calculus) and angular velocity is the speed and direction (in this context clockwise or anticlockwise) in which an angle is changing. That angle can be that of a body rotating on its centre of mass, or that of a body in circular motion about a point (as we have here). So what velocity is to position, angular velocity is to angle and what acceleration is to velocity, angular acceleration is to angular velocity.
So a “normal” to a line is a direction which is perpendicular to that line. (In 3D you can also get a normal to a face, which is a direction perpendicular to that face). Example:
http://images.tutorvista.com/cms/images/67/perpendicular-line-definition.png
So if we have a rope with a guy on the end, then the normal to the rope is the tangent to the circular path the guy is going to swing in. Ie it is the direction he will travel in at that moment in time which we call the tangential velocity. (aT in the previous post stood for tangential acceleration).
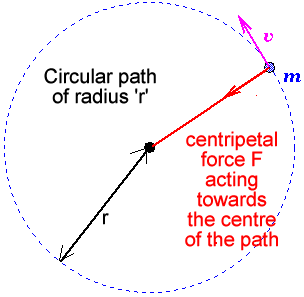
In that diagram, v is the tangential velocity. Ignore the centripetal force thing.
So now, my code example. I’m going to write a SwingingBody class with an update() method which takes the delta time for the current frame as a parameter. It uses euler integration to compute the current angle of swing. Things I’m leaving out are a way to render it and any collision detection/handling.
public class SwingingBody {
public static final float g = 9.81; //Constant for average gravitational acceleration at the surface of the Earth.
private float angle = 0; //The angle between the rope and the horizontal.
private float av = 0; //angular velocity
private float r; //The radius of the swing - the length of the rope.
public void update(float delta) {
float aa = g * GMath.cos(angle) / r;
av += ( aa * delta );
angle += ( av * delta );
}
}
Three notes about this code:
-
I have used a function called GMath.cos(), this is meant to be a regular cos function accept that it takes a float as a parameter and returns a float as well. I don’t know what framework you’re using so I just used my own framework.
-
Generally we work with delta in milliseconds, however the constant for g I have given is in metres per second squared. So you must either divide delta by 1000 to get it in seconds or divide g by 1000,000 to get it in metres per millisecond squared.
-
Sorry about the fancy definition for g, I’m a physicist at heart.
Hope this helps.
Quew8